Sense Hat Raspberry Pi Zero

This example shows you how to read the sensor values, read the position of joystick and control the LED Matrix on Raspberry Pi™ Sense HAT using MATLAB™ Support Package for Raspberry Pi Hardware.
Introduction
Note: The Raspberry Pi Sense HAT is compatible with the Raspberry Pi 3, Raspberry Pi 2, Model B+, and Model A+, but NOT the earlier 26-pin models of Raspberry Pi 1 Model B & A's. Pi not included! This forum post mentions getting the sense hat to work with the Pi Zero and older versions of the Raspbian software, but it looks like this has. The Sense HAT, which is a fundamental part of the Astro Pi mission, allows your Raspberry Pi to sense the world around it. In this project, you will learn how to control the Sense HAT’s LED matrix and collect sensor data, and you will combine these ideas in a number of small projects. Raspberry Pi Zero W. Single-board computer with wireless and Bluetooth connectivity. The Sense HAT is an add-on board for Raspberry Pi.
The Raspberry Pi Sense HAT is an add-on board that includes an 8X8 RGB LED matrix, a five-position joystick and the following sensors:
Humidity sensor
Pressure sensor
IMU sensor (Accelerometer, Gyroscope, and Magnetometer).
In this example you will learn how to create a sensehat
object to connect to the Raspberry Pi Sense HAT, read sensor values, read the position of the joystick and control the LED matrix.
Prerequisites
We recommend completing Getting Started with MATLAB Support Package for Raspberry Pi Hardware example
Required Hardware
To run this example you will need the following hardware:
Connect Sense HAT
Attach the Sense HAT to the Raspberry Pi expansion header. Make sure that you power down your Raspberry Pi before attempting to attach the Sense HAT.
Create a Sense HAT object
Create a Sense HAT object by executing the following on the MATLAB prompt.
mysensehat
is a handle to a sensehat object.
Let us read the temperature from Sense HAT.
Reading Environmental Sensors
Sense HAT includes humidity and pressure sensors which measure the temperature, the pressure and the humidity. Let's read the value of humidity and pressure measured by these sensors.
Temperature can be read from either the humidity sensor or the pressure sensor. To read the temperature from the humidity sensor, execute the following on the MATLAB prompt:
To read the temperature from the pressure sensor, execute the following on the MATLAB prompt:
By default, the temperature is read from the humidity sensor. Hence, readTemperature(mysensehat)
is same as readTemperature(mysensehat,'HumiditySensor')
.
Read the IMU sensor.
Sense HAT has an IMU sensor which consists of an accelerometer, a gyroscope and a magnetometer. The IMU sensor measures acceleration, angular velocity and magnetic field along the X, Y and Z axis. To read the acceleration, execute the following on the MATLAB prompt:
Supreme ruler 2020 mod. readAcceleration
returns a 1-by-3 vector of acceleration values measured along the X, Y and Z axis.
To read the angular velocity, execute the following on the MATLAB prompt:
readAngularVelocity
returns a 1-by-3 vector of angular velocity values measured along the X, Y, and Z axis.
To read the magnetic field, execute the following on the MATLAB prompt:
readMagneticField
Avid composer 6 keygen crack. returns a 1-by-3 vector of magnetic field values measured along the X, Y, and Z axis.
Read the state of the joystick
Sense HAT has a 5 position mini joystick. Let us read the position of the joystick.
readJoystick
returns the a value between 0 to 5 depending on the position of the joystick.
0 - Not pressed
1 - Centre
2 - Left
3 - Up
4 - Right
5 - Down
Raspberry Pi Rf Hat
The numerical values representing joystick positions are ordered with respect to the reference position of the Sense HAT. The HDMI port of the Raspberry Pi board facing downwards is the reference position.
You can monitor a particular joystick position by executing the following on the MATLAB prompt:
readJoystick(sensehatObj,position)
will return a logical one if the joystick is in the specified position and zero otherwise.
Use the LED matrix
Sense HAT has a matrix of 8x8 RGB LEDs. You can set the color of a particular pixel, display an image or display a scrolling message on the LED matrix. Let us set the color of the pixel in the 3rd row, 6th column to cyan.

Observe that the pixel in the specified location is now cyan in color.
Mac App Store is the simplest way to find and download apps for your Mac. To download apps from the Mac App Store, you need a Mac with OS X 10.6.6 or later. Jan 02, 2020. Apr 06, 2020. Jul 20, 2017. How to download mac apps. Jan 17, 2020.
The rows and columns are numbered 1 to 8. Pixel at location [1 1] is at the top left corner of the LED Matrix. The location is with respect to the reference position of the Sense HAT. The HDMI port of the Raspberry Pi board facing downwards is the reference position.
Color of a pixel can be specified by name. The supported colors are:
Red
Blue
Green
Cyan
Magenta
Yellow
White
You can also specify any other color by using a 1x3 array of R, G, B values. For example, setting the pixel to [255 192 203], would set it to pink. Now, let us display an image on the LED matrix.
The image must be an NxMx3 array of uint8 values. You can change the orientation of the displayed image by executing the following on the MATLAB prompt:
The Orientation of the image can be set to 0, 90, 180 or 270 degrees. These are defined with respect to the reference position of the Sense HAT. The HDMI port of the Raspberry Pi board facing downwards is the reference position.
LED Matrix can be used to display messages in the form of a scrolling text. The text scrolls from left to right. You can change the orientation of scroll by setting the 'Orientation' property of LEDMatrix. Let us display 'Hello World' on the LED Matrix.
By default, the text scrolls with a scrolling speed of 0.1 second and the color of the text is red. To increase the scrolling speed to 0.05 second, execute the following on the MATLAB prompt:
In order to set the text color to yellow, execute the following on the MATLAB prompt:
Similarly, in order to set the background color to white, execute the following on the MATLAB prompt:
You can rotate the text by specifying the orientation for the display. Let us specify orientation as 90 and display the text message.

Now, let us try to display a numeric value on the LED matrix. Read the value of temperature and display it on the LED matrix.
To clear the LED matrix, execute the following on the MATLAB prompt.
Summary
This example introduced the workflow for using MATLAB Support Package for Raspberry Pi Sense HAT.
Installation
In order to work correctly, the Sense HAT requires an up-to-date kernel, I2C to be enabled, and a few libraries to get started.
Ensure your APT package list is up-to-date:
Next, install the sense-hat package which will ensure the kernel is up-to-date, enable I2C, and install the necessary libraries and programs:
Finally, a reboot may be required if I2C was disabled or the kernel was not up-to-date prior to the install:
Hardware
The schematics can be found here.
Software overview
After installation, example code can be found under /usr/src/sense-hat/examples
.
These can be copied to the user's home directory by running cp /usr/src/sense-hat/examples ~/ -a
.
The C/C++ examples can be compiled by running make
in the appropriate directory.
The RTIMULibDrive11 example comes pre-compiled to help ensure everything works as intended. It can be launched by running RTIMULibDrive11
and closed by pressing Ctrl+c
.
Python sense-hat
sense-hat
is the officially supported library for the Sense HAT; it provides access to all of the on-board sensors and the LED matrix.
Complete documentation can be found at pythonhosted.org/sense-hat.
RTIMULib
RTIMULib is a C++ and Python library that makes it easy to use 9-dof and 10-dof IMUs with embedded Linux systems. A pre-calibrated settings file is provided in /etc/RTIMULib.ini
, which is also copied and used by sense-hat
. The included examples look for RTIMULib.ini
in the current working directory, so you may wish to copy the file there to get more accurate data.
Other
LED matrix
The LED matrix is an RGB565 framebuffer with the id 'RPi-Sense FB'. The appropriate device node can be written to as a standard file or mmap-ed. The included 'snake' example shows how to access the framebuffer.
Joystick
The joystick comes up as an input event device named 'Raspberry Pi Sense HAT Joystick', mapped to the arrow keys and Enter
. It should be supported by any library which is capable of handling inputs, or directly through the evdev interface. Suitable libraries include SDL, pygame and python-evdev. The included 'snake' example shows how to access the joystick directly.
Calibration
Taken from this forum post.
Install the necessary software and run the calibration program as follows:
You will then see this menu:
Press lowercase m
. The following message will then show; press any key to start.
After it starts, you will see something similar to this scrolling up the screen:
Focus on the two lines at the very bottom of the screen, as these are the most recently posted measurements from the program.Now you have to move the Astro Pi around in every possible way you can think of. It helps if you unplug all non-essential cables to avoid clutter.
Try and get a complete circle in each of the pitch, roll and yaw axes. Take care not to accidentally eject the SD card while doing this. Spend a few minutes moving the Astro Pi, and stop when you find that the numbers are not changing anymore.
Now press lowercase s
then lowercase x
to exit the program. If you run the ls
command now, you'll see a new RTIMULib.ini
file has been created.
In addition to those steps, you can also do the ellipsoid fit by performing the steps above, but pressing e
instead of m
.
Raspberry Pi Zero Wiki
When you're done, copy the resulting RTIMULib.ini
to /etc/ and remove the local copy in ~/.config/sense_hat/
:
You are now done.
Updating the AVR firmware
..
EEPROM data
These steps may not work on Raspberry Pi 2 Model B Rev 1.0 and Raspberry Pi 3 Model B boards. The firmware will take control of I2C0, causing the ID pins to be configured as inputs.
Enable I2C0 and I2C1 by adding the following line to
/boot/config.txt
:Enter the following command to reboot:
Download and build the flash tool:
Reading
EEPROM data can be read with the following command:
Writing
Raspberry Pi
Please note that this operation is potentially dangerous, and is not needed for the everyday user. The steps below are provided for debugging purposes only. If an error occurs, the HAT may no longer be automatically detected.
Raspberry Pi Hat List
Download EEPROM settings and build the
.eep
binary:Disable write protection:
Write the EEPROM data:
Re-enable write protection:

Sound Frequency Meter Software

Feb 23, 2015 Calculate: Frequency Analyzer uses the algorithm called the fast Fourier transform or FFT. In this way, it calculates the digital signals that are the components of a sound. The user is allowed to increase the speed of the computer's sound card to get an accurate graph. Pros Frequency Analyzer can be used and downloaded for free. This sound meter also has internal memory that can record and store 4700 data points which can be imported into the included software for review. Other great features include; automatic display back-light, AC/PWM Outputs, Adjustable Sampling rate preset to 8x/second, Low Battery Indicator, Frequency response range of 31.5hz 8.5KHz.
A SIMPLE FREQUENCY METER
(2010-2018)
The frequency meter. The program is written in the programming language Python.
Therefore, it is simple to adapt it to your own requirements.
No primitive frequency counters with leds anymore! Throw them away! We replace these simple frequency counters by a real display on the PC. That looks much nicer, is not so primitive and the hardware is simpler too, only 1 IC! And the frequency is much simpler and nicer readable and more accurate!
The idea is very simple and applied more often. We divide the RF signal with a prescaler to audio frequencies. This audio signal is connected to the soundcard of the PC and we measure the audio frequency. Then this frequency is multiplied with the prescaler value and.. we do have the RF frequency!
When you press 'Stop', then the current settings are stored in the file 'recent.jpg' and loaded when you run the program the next time.
The hardware, a very simple box.
Sound Frequency Meter Software For Windows 10
A frequency counter counts during a certain time the number of periods. This frequency counter works differently. Of a certain number of periods, the exact time is measured.
The resolution of this time measurement depends on the sample rate of the soundcard. The resolution of the time measurement is plus or minus 1 sample. But by adding one extra resistor and one extra capacitor, the accuracy can be improved by a factor 20x! And with fast measuring times of 0.2 seconds, that you want to use for tuning a receiver, we still do have a good accuracy.
When the slope is reduced by means of an RC network, we do also have
amplitude information and we can calculate where between both
samples the zero crossing is.
With a square wave signal from the prescaler, we do not know where exactly between the two audio samples of the soundcard the zero crossing is. The first sample is always +5 volt and the next sample 0 volt. So the accuracy of the time measurement is always plus or minus 1 sample. But when we could calculate where exactly the zero crossing is between the two samples, we can also measure with fractions of samples and then the accuracy of the time measurement is much better! And that is possible! When the slope is reduced by means of an RC network, we also have amplitude information and then we can determine on which place between both samples the zero crossing is! Is U2 smaller than U1, then the zero crossing is closer to S3 than to S2. And with the ration U1:U2, we can calculate exactly where the zero crossing is!
Prescaler upto at least 30 MHz with built in frequency reference.
With an extra prescaler, you can expand the range upto a few GHz.
big diagram
At the input, you will find a limiter to prevent damage. It consists of a resistor R1 of 1k ohm and two diodes connected anti parallel. With S1 in the lower position, the RF signal goes to the input of the 74HC4060. This IC divides the RF signal by 4096 to an audio frequency. At the output of the 74HC4060, you can find the RC network of 10k ohm and 10nF to reduce the slope. And the resistor network of 22k and 1k does attenuate the audio level so that the soundcard is not overdriven.
The two resistors of 1M are for the correct DC setting of the input. The capacitor of 10nF does filter the AC component. Otherwise there is feedback that reduces the gain at low frequencies.
With S1 in the upper position, the 74HC4060 does work as a crystal oscillator, and then you have a reference frequency for the calibration of the soundcard. It is also possible to use a different crystal of course. You do not have to adjust the frequency. My copy has a frequency of 4095.754 kHz and I enter that frequency during the calibration.
The circuit is supplied via the USB port. Go to the shop for a cheap USB cable, cut of the right plug, find the right wires and you do have a 5 volt supply. As the supply voltage is 5 volt, you can also use the better available 74HCT4060 instead of the 74HC4060. There is an extra output in the circuit for a 4 kHz / 5 volt calibration signal for a simple oscilloscope working with the soundcard.
Frequency (MHz) | Sensitivity with R1=120 (mV RMS) | Sensitivity with R1=1k (mV RMS) |
0.03 0.1 1 10 20 30 50 80 100 120 | 15 10 3 10 25 30 100 150 200 500 | 15 10 5 20 50 100 300 1000 - - |
Sensitivity of the simple prescaler.
AccuracyTests were performed with the internal soundmodule of a laptop and with a simple, exteran USB audio device. The calibration signal of 1 MHz comes from aGPS locked module.
The internal sound module gave a deviation of +20Hz. The USB audio device had a deviation of -75Hz.
After calibration, there was a variation of 1 to 2 Hz in the measured values. This is tested with a measuring time of 1 second.
Setting of the sound module
Set the sample rate to the default value of your operating system. Usually that is 44100 Samples/sec.
You can connect the prescaler to the line input or to the microphone input of the sound card. When you use the microphone input, you must switch off the extra 20 dB amplification. Adjust the input level so that this level is well below the maximum of 100%, for example 5% -20%. With the mouse you can adjust the volume control of the sound module. How this has to be done, depends depends on the used sound module, your operating system and its version.
Calibration
There are two possibilities for the calibration:
Possibility 1: Select 'Prescaler' and enter manually the prescaler value.
The prescaler value has to be chosen so that the exact value of the frequency is displayed. So not 4096, but for example 4096.0235.
Possibility 2: Connect the frequency meter to a reference frequency and do a measurement with a long measurement time of 5 seconds.
Click 'Stop' to stop the measurement. Select 'Prescaler' but press 'Cancel' instead of to enter a prescaler value.
Answer the question 'Calibrate with measured frequency' with 'yes' and enter the reference frequency in Hz. The exact prescaler value is calculated automatically. You can store it together with the other settings like offset frequency. For the calibration, you can use the in the prescaler built-in crystal oscillator of course.
Other applications
Many amateurs circuits do have a frequency stabilizer (for example a Huff & Puff), with which the VFO signal is already divided by a prescaler (for example a 74HC4060) to audio frequencies. The only things you have to add is the simple RC network consisting of 3 resistors and 1 capacitor and a cable with plug. The IF frequency can be programmed as a frequency offset and we do have a very nice frequency display. Very often, the PC is already connected to the receiver to decode digital modes. A switch for 'audio out' or 'prescaler out' is then very handy.
Barefoot Technology!
Bare feet are the best shoes when working with electronics.
No problems with electrostatic electricity!
SOFTWARE
User Guide Episode 6.5 User Guide for Mac 161756 May 20, 2015. Telestream episode 6 for mac. We would like to show you a description here but the site won’t allow us. I have two iMacs running different versions of the MacOS, each with a different version of Episode: iMac from 2011 Max OSX 10.6.8 (Snow Leopard) Episode Version 6.3.1.23 iMac from 2017: MacOS 10.13.
Before you use this program, you have to install Python. That is very simple. But read first something about Python by clicking the following link:As the source code of Python is written in ASCII, it is very simple to modify the program to you own requirements. Think for example about the size of the screen, the colors etc.
Required Python version:
Apple app store online.
- Python version 3
Light Frequency Meter
Required external modules (site-packages for the correct Python version!):Sound Frequency Meter In Hz

Audio Frequency Meter
- numpy; pyaudio
Sound Frequency Meter Software

Sound Frequency Meter Software Reviews

Fl Studio 12 R2r Torrent
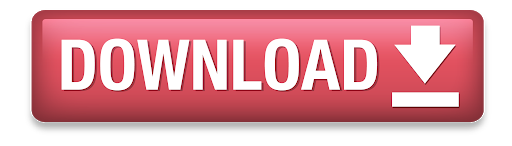
Jan 25, 2018 FL Studio 12.5.1.165 (2018) Full Cracked Version. FL Studio 12.5.1.165: is a complete software music production environment or DAW (Digital Audio Workstation) representing more than 14 years of innovative developments and our commitment to Lifetime Free Updates. Everything you need in one package to compose, arrange, record, edit, mix and master professional quality music. Image-line FL. Sep 28, 2019 FL Studio 12.4.2 Producer Edition MAC & Windows Crack (Fruity Loops all editions Universal Crack) FL Studio 12: is a famous and extremely powerful music editing tool. The program was formerly known as Fruity Loops, but recently it has gone through a transformation from a MIDI sequencer to professional audio editing software.


- Sep 29, 2019 FL Studio v.20.0.3.532 Incl.Patch and Keygen-R2R deepstatus.torrent.
- Sep 04, 2020 So, FL Studio 20 Keygen is to use a digital audio workstation. Moreover, you can change the pitch or pitch-shifting or time-stretching of audio. Divide your track into the slice to produce a new track using the slices. FL Studio 20 Crack Reddit: Comparing to other professional software FL Studio 20 Registration Key is quite simple.
We know that we are surrounded by computer technology. The computer has replaces all the physical things that a human used to produce something. As above mentioned, in the music industry computer also bring the revolution. All the musical instruments are replaced by some music production applications. So, if you are looking for the best musical software that provides you with a complete environment for music production. Then, Fl Studio 20.7.1.1773 Crack Reddit Download developed by Image-Line is the best result of their search. It provides a complete environment for music producers. Which allows recording multi-track at the one time.
Best price on mac. We can say that it is the Digital Audio Workstation. You can do multiple tasks from it using its numerous built-in powerful tools. You can record multiple tracks, mix and sequence them together to generate new music. FL Studio 20 Cracked is for the professional or amateur editors who can use this to produce high-quality music tracks. It enables you to take advantage of the mixer, advanced MIDI, DX, and Rewrite support.
The multiple audio track recording feature is the best feature. Users can use this feature to record all the tracks simultaneously that our player supports. So, record your music with its realtime recording tool. Other software are providing this feature to its user as they are developing the software with the old technology.
Apply Different EFfects to your Music:
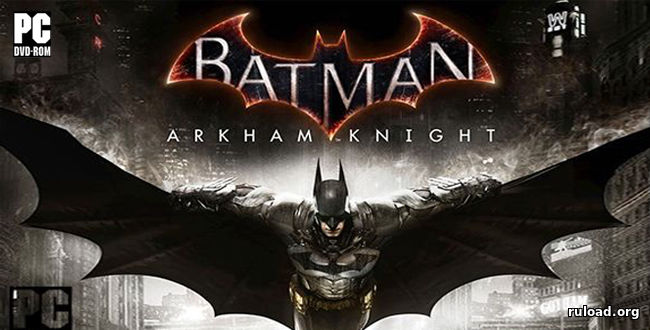
The amazing feature that is mentioned above is that the multiple track recording. That means now the user can record the track from multiple recording devices continuously. There is no need to wait for the one recording to complete recording and then record from the other device. This is time-consuming and didn’t provide the user with realtime a good MIDI software. FL Studio 20 RegKey Crack fulfills the requirement of all the users so that they can get the full benefit from the Image-Line products. This company is famous because of its stunning products for the music industry. Therefore, this software is also getting the no 1 place among all the music composer applications.
Additionally, you can add a different effect to your tracks. if you want to edit music. you just have to drag & drop to the music timeline. it will auto-detect the different patterns of music using pattern technology. the pattern technology is the most powerful technology in the music editing software. Almost all software use this technology. So, after detecting the patterns it allows you to make different effects that you want. So, FL Studio 20 Keygen is to use a digital audio workstation. Moreover, you can change the pitch or pitch-shifting or time-stretching of audio. Divide your track into the slice to produce a new track using the slices.
FL Studio 20 Crack Reddit:
Comparing to other professional software FL Studio 20 Registration Key is quite simple. Complex tasks or editing can be easily done using its simple user-friendly interface. The interface can easily be personalized as the needs of the different users. Like if a mixer uses this then he can show up all the mixing tools on the interface. Also, you can capture different inputs from keyboards, drum pads, or MIDI. All these devices can be easily controlled differently.
Additionally, you can do remix the audio tracks easily With FL Studio 20 RegKey Free. Then you can add real-time effects to your tracks. The effects like reverb, delay, and filtering easily applied to your tracks. Moreover, you can mix up different tracks to obtain high new real-time music.
The ability of plugins enhances performance. Different plugins for specific tasks are available on the internet. You can download and complete your work efficiently.
FL Studio 20 Crack Reddit Download:
Without having the ability to add the plugin in any multimedia software will be a drawback of that software. Therefore, that should be overcome. In the producer edition, the support for adding the plugin according to the user’s choice has been added. Now the user can add the plugin that they are really needed. He can get rid of all the unnecessary plugins that may slowdowns the computer.
Conclusion: After reading the key features of FL Studio 20 RegKey Crack. I can recommend you to use this full feature riched software. It is the fully functional software for the Music editors. They can generate music fast even they can think. We can say that it is the Numerous gift for music editors to use. It is suitable for all types of users that belong to the music industry. They will find this software fully userfriendly and easy to use. Also, they can watch the video tutorials from the YouTube to get all the information about all the tools. Therefore, Download Free FL Studio Cracked Registration Key from the Given Below Link.
Rich Key Features:
- Mixing:
- Multi-tracks can mix up to each other
- Effects:
- Real-time effects can also apply to the tracks
- Sequence or pattern:
- Patterns of tracks are generated to apply filters to music
- Plugins:
- Add plugins for different tasks
- Interface:
- User-friendly interface that can everyone use
- Multiple MIDI:
- You can edit more than one midi-ins at one time
- Support Multiple OS:
- FL Studio 12 Producer Edition Torrent is available for MacOSX, Apple iPhone, iPod
- Browser:
- Built-in Search engine to search for tracks
- Playlist:
- Automatically playlists generated
- Powerful tools:
- All audio powerful tools are included in the latest version
Also, See:
What’s new in FL Studio Torrent Download:
Version 20.7.1.1773:
Fl Studio 12 R2r
- The latest version supports iPhone and iPod
- New Plugin ‘FLUX’ has been added to FL Studio reg key
- Fire Extension pack is free available on the official website
- Adding an instrument or audio track doesn’t switch from pattern to song mode anymore
- “Separate from instrument track” is renamed and can also link a channel to a new instrument track
- Removed (delete) presets from the default plugin databases as there are dedicated options for that
How To Get FL Studio 20.7.1.1773 Crack?
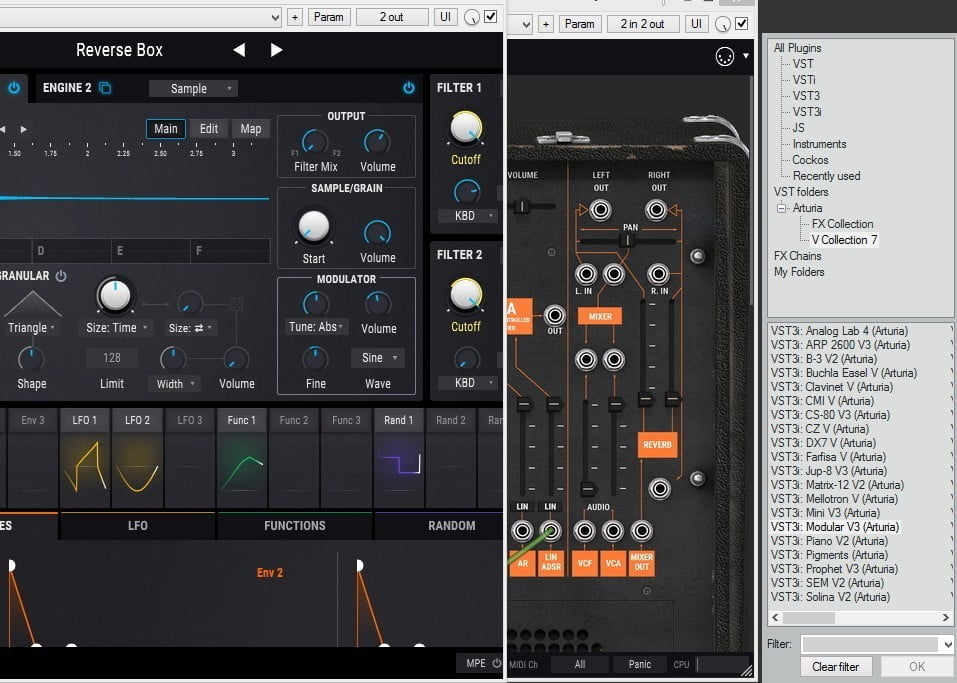
- Download the Zip file from any downloading link or watch the video to get link
- Extract the file that you have downloaded from our secure servers
- Install the Trial Version of this software to get all the files from the official website
- Disconnect from the internet as it will prevent to register the software for lifetime
- Open the Crack Folder that is placed in the Zip file
- Click on the Crack File to get the Image-Line products for the lifetime
- Block the Firewall if it interrupts while Cracking
- Now, Enjoy the FL Studio 20 Crack Reddit Latest Version
Fl Studio 12 Torrent Download
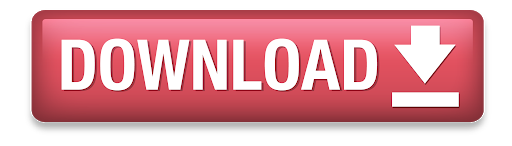
Best Price On Mac
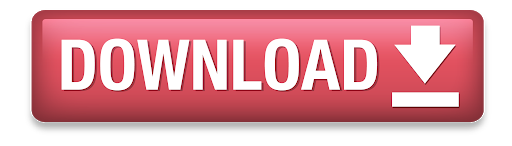
Save on the new MacBook Air and the new 13-inch MacBook Pro in our Education Store. Shop education pricing
New27-inch modeliMac
MacBook Air
13-inch modelMacBook Pro
$699.99 Your price for this item is $699.99 Sold Out Apple - 27' iMac® with Retina 5K display - Intel Core i5 (3.7GHz) - 8GB Memory - 2TB Fusion Drive - Silver. Consider the following price ranges when budgeting for a new MacBook. Realmac software rapidweaver web design software for mac. In the $1,000 to $1,500 range, you’ll find entry-level and mid-range MacBooks in their base configurations. Keep in mind that crucial upgrades can drive prices up quickly, so when you’re window-shopping, be sure to mentally tack on the cost of upgrades like additional RAM. Sep 01, 2020.
Which Mac notebook is right for you?
- 13.3-inch Retina display1
- Up to 4-core Intel Core i7 processor
- Up to 16GB memory
- Up to 2TB storage2
- Up to 11 hours battery life3
- Touch ID
- Backlit Magic Keyboard
Best Price On Macbook Pro
- 13.3-inch Retina display1
- Up to 4-core Intel Core i7 processor
- Up to 32GB memory
- Up to 4TB storage2
- Up to 10 hours battery life3
- Touch Bar and Touch ID
- Backlit Magic Keyboard
- 16-inch Retina display1
- Up to 8-core Intel Core i9 processor
- Up to 64GB memory
- Up to 8TB storage2
- Up to 11 hours battery life3
- Touch Bar and Touch ID
- Backlit Magic Keyboard
macOS Big Sur
Doing it all,
in all new ways.
Free delivery
Get free shipping direct to your door. See checkout for delivery dates.
Find a reseller

Apple has hundreds of authorized resellers. Chances are there’s one near you.
macOS Catalina
The power of Mac.
Taken further.
Dedicated apps for music, TV, and podcasts. Smart new features like Sidecar, powerful technologies for developers, and your favorite iPad apps, now on Mac.
macOS
macOS is the operating system that powers every Mac. It lets you do things you simply can’t with other computers. That’s because it’s designed specifically for the hardware it runs on — and vice versa.

iCloud
iCloud safely and securely stores your photos, videos, documents, messages, music, apps, and more — and keeps them updated across all your devices. So you always have access to what you want, wherever you want it.
Built-in Apps
Powerful creativity and productivity tools live inside every Mac — apps that help you explore, connect, and work more efficiently.
Keep your growing library organized and accessible. Perfect your images and create beautiful gifts for sharing. And with iCloud Photos, you can store a lifetime’s worth of photos and videos in the cloud.
Tell stories like never before. A simple design and intuitive editing features make it easy to create beautiful 4K movies and Hollywood-style trailers.
The easiest way to create great-sounding songs on your Mac. With an intuitive interface and access to a complete sound library, it’s never been easier to learn, play, record, and share music like a pro.
Best Price On Mac Air
This powerful word processor gives you everything you need to create documents that look beautiful. And read beautifully. It lets you work seamlessly between Mac, iOS, and iPadOS devices. And work effortlessly with people who use Microsoft Word.
Create sophisticated spreadsheets with dramatic interactive charts, tables, and images that paint a revealing picture of your data. Work seamlessly between Mac, iOS, and iPadOS devices. And work effortlessly with people who use Microsoft Excel.
Bring your ideas to life with beautiful presentations. Employ powerful tools and dazzling effects that keep your audience engaged. Work seamlessly between Mac, iOS, and iPadOS devices. And work effortlessly with people who use Microsoft PowerPoint.
Safari has innovative features that let you enjoy more of the web. In even more ways. Built-in privacy features help protect your information and keep your Mac secure. An updated start page helps you easily and quickly save, find, and share your favorite sites. And Siri suggestions surface bookmarks, links from your reading list, iCloud Tabs, links you receive in Messages, and more.
Pro Apps
For professionals ready to push their creativity, these industry-leading apps offer maximum control over editing, processing, and output of music and film.
Logic Pro puts a complete recording and MIDI production studio on your Mac, with everything you need to write, record, edit, and mix like never before. And with a huge collection of full-featured plug-ins along with thousands of sounds and loops, you’ll have everything you need to go from first inspiration to final master, no matter what kind of music you want to create.
Take your Mac to the stage with a full-screen interface optimized for live performance, flexible hardware control, and a massive collection of plug-ins and sounds that are fully compatible with Logic Pro X. Avid composer 6 keygen crack download.
Built to meet the needs of today’s creative editors, Final Cut Pro offers revolutionary video editing, powerful media organization, and incredible performance optimized for Mac computers and macOS Catalina.
Motion is a powerful motion graphics tool that makes it easy to create cinematic 2D and 3D titles, fluid transitions, and realistic effects in real time.
Add power and flexibility for exporting projects from Final Cut Pro. Customize output settings, work faster with distributed encoding, and easily package your film for the iTunes Store.
The Mac App Store features rich editorial content and great apps for Mac. Explore the Mac App Store
Apple TV Plus
Get one year of Apple TV+ free
when you buy a Mac.4
Apple Arcade
Calling all players.
Hundreds of worlds. Zero ads.
- Try it free5
Mac for Education
Power to make big things happen in the classroom.
Mac for Higher Education
Ready for everything college has to offer.
Mac for Business
Get the power to take your business to the next level.
Upgrade to start your free trial.
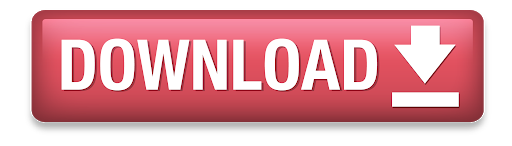
Apple Mac Os 10.13 Download

- Aug 04, 2017.
- At this year’s WWDC 2017 conference, Apple announced macOS 10.13 High Sierra to the world. This latest version of the operating system that runs on Macs and MacBooks comes with a number of great.
- May 28, 2018.
- Mar 23, 2020.
The macOS Mojave was the fifteenth release from Apple for their macOS family and was made available to the public on the 24th of September in 2018. The macOS Mojave 10.14.1 (the updated version) was released on the 30th of October of the same year with a couple of new updates.
It succeeded Mac OS High Sierra 10.13 which was released on the 25th of September in 2017 and was preceded by the macOS Catalina 10.15 which was released on the 7th of October in 2019. This macOS is known to be the last one developed by Apple to support 32-bit applications as macOS Catalina only supports 64-bit applications.
Download Latest Version: macOS Catalina 10.15 ISO & DMG Image
License
Official Installer
File Size
5.6GB / 5.9GB
Jan 04, 2018.
Language
English
Developer
Apple Inc.
Tutorial: How to Install Mac OS on Windows PC using Virtual Machine.
Redshift 2.6.41 crack. There were a large number of new features and additions introduced in macOS Mojave that made it successful. In particular, Dark Mode, Stacks and the improved App Store received fine praise. Additions to the Finder and Screenshot features helped improve their ability to serve the needs of the users greatly. The focus on strong security for the user’s information was also viewed favourably.
At the same time, the macOS received criticism for its beta performance issues. Not all the features implemented on the system were completely developed either. Despite these shortcomings, macOS Mojave was seen as a powerful step forward for Apple towards its future products.
Productive Features of macOS Mojave 10.14.1
Here are some of the features that were introduced in Mojave and some of the pre-existing ones that have been reworked:
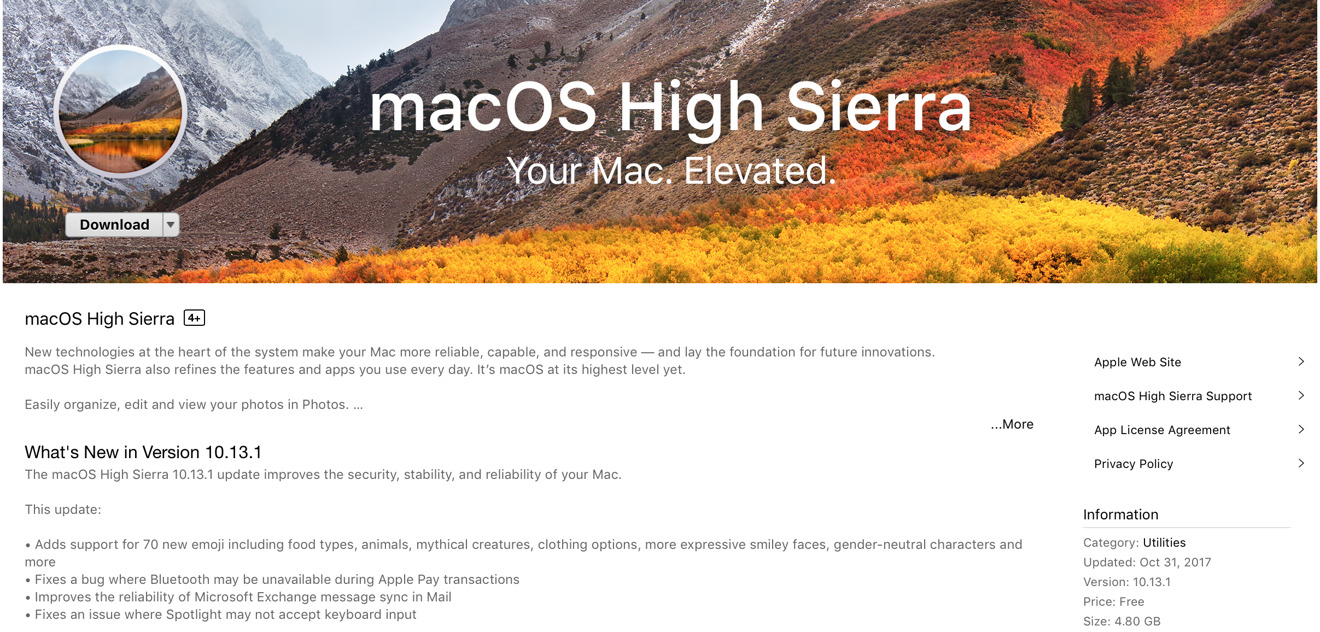
- Finder Quick Actions- This is a useful feature that will allow users to create a PDF or rotate an image without the use of an app. The user can explore the kind of actions that they can perform by checking out the Quick Actions menu.
- Finder Preview Panel- Like before,this feature allows the user to have a small glance into a document or an image without actually opening it. Additionally, users will now be able to be aware of details about the document or images such as the date of document creation or the kind of lens used for taking a photo.
- Quick Look- A pre-existing app on macOS Sierra, Quick Look allows users to see files, folders or photos without even opening them. With the new Markup feature, photos and PDFs can now be cropped or rotated. Text can also be added to them.
- Security improvements- Ad trackers that constantly bombard users with advertisements when they show interest in a product will now be blocked by the macOS. The macOS also now asks the user for permission for apps to use their Mac’s microphone and camera. Permissions will also be asked for other things such as browser data and message history.
- Safari Favicons- Since favicons were not available in Safari before, most people preferred to use Google Chrome. Now, favicons are available in Safari which means that users will be able to enjoy what they have been desperately waiting for. With the aid of favicons, users will now have much greater ease identifying their tabs without having to open them. This may be a small addition but it has made many users incredibly ecstatic.
- Dock- This is a pre-existing feature that can be used to get to apps and features that users are prone to access on a daily basis. In macOS Mojave, the Dock will show users up to 3 apps that they have used recently. Since not everyone is a fan of this feature as some find it annoying, they can turn it off if they wish.
- Facetime- Users can now chat with up to 32 people at the same time. If there is a group call happening, each participant can join in whenever they want during the active duration of the call. Group messages can also be sent in Facetime.
- App Store- The App Store has received many new additions to its already impressive set of features. The newly introduced Discover tab helps users find apps that they may have missed out on before, based on their preferences. The Create app will help users who are interested in producing content through methods like video editing and filmmaking.
- Dark Mode- By using Dark Mode, users will now be able to switch between Light Mode as well as Dark Mode. Using this new mode, users can protect their eyes from strain when they are working at night. The blue light that is emitted from the Mac screen is not good for the user’s eyes and Dark Mode helps reduce it greatly. The mode makes it easier to read text on the screen if there is good contrast between the screen and what the user is reading. Using Dark Mode can also help save battery life.
- Continuity Camera- If the user’s Mac and iOS devices are in close proximity to each other and both have their Wi-Fi and Bluetooth turned on, this feature can be used. It allows users to have whatever they scan or take a picture of on their iOS device be immediately available for display on their Mac. This feature can save a lot of time that would normally be taken in transferring photos or documents from iOS devices to the Mac the manual way.
- Dynamic Desktops- This feature changes the wallpaper of Mac’s screen according to the time of day. In order to use this feature, the user must have their Location Services enabled as the feature needs this information in order to be able to draw a match between the lighting outside in the user’s location with the wallpaper that they have on their screen.
- New ported apps- A few apps have been ported to the macOS Mojave from the iOS, namely News, Stocks, Voice Memos, and Home. News is an incredibly informative app that will provide users with updates and news pages from the world. With Home, users will now be able to connect their Mac with their HomeKit accessories which help them control their environment(light-bulbs, smart door locks, etc). If you wish to use your voice to record yourself singing or create voice memos, Voice is the app for you. Users can also sync their voice memos with all their iOS devices. Stocks will help the user get all of the financial information and stock market details that they need, complete with excellent diagrammatic representations with the appropriate statistical data attached.
- Stacks- Having a messy desktop can be excruciating to deal with it, especially for those who try to organize the multitude of files on their Desktop and end up failing. With the Stacks option, those worries can now be put to rest. Users will now be able to neatly arrange their files into neat stacks according to their wishes. There are different fields according to which the stacking can take place depending on the user’s preference such as the date the file was last modified or the date that the file was created. Stacks has been praised as one of the brightest additions introduced in macOS Mojave.
- Screenshot markup- There are many more options to take screenshots in macOS Mojave. These include being able to screenshot the entire screen, a specific window, or a specific area. The user is also able to screen record the entire screen or a specific part of it according to their preferences(for video purposes). They can also add text, shapes, or color to their screenshot if they wish.
- Siri- Siri has always been an important part of the Apple family and a great virtual assistant to users. In macOS Mojave, Siri is now able to control HomeKit devices and can also assist the user in finding their saved passwords.
- Updates- Normally, updates are performed through the App Store. In macOS Mojave, there is a Software Updates panel under System Preferences that has been designed especially for this purpose.
- Inclusion of new emojis- This may not seem like addition of great importance but the excitement that comes with it is nothing short of huge. More than 70 new emojis have been introduced to Apple’s emoji family.
- Emojis in the mail- Emojis can now be included in the user’s mails. There is a convenient shortcut available that the user can utilise in order to do this.
How to download the macOS Mojave ISO and DMG files
There are certain conditions that have to be satisfied before proceeding with the download. Using a verified link online, the user should download VirtualBox or VMWare. After this is done, the user should proceed to the Terminal and type in the required commands that come under each of the specified steps below:
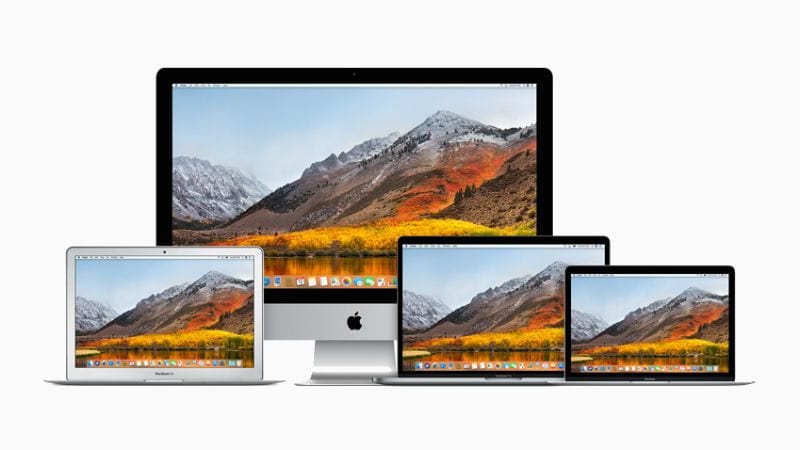
- Creating a virtual disk for the installation media.
- Mounting the virtual disk.
- Writing the installer to the mount point.
- Unmounting the installer app.
- Converting the DMG file to the ISO format.
- Changing the file extension to an ISO file.
Requirements for macOS Mojave 10.14.1
The user’s Mac must have a minimum of 2 GB of RAM and 12.5 GB of available storage space in case they are upgrading to macOS Mojave from any one of its predecessors from OS X El Capitan and onwards. It will require 18.5 GB of available storage space if the upgrade is for an OS that is or precedes OS X Yosemite.
Steps to download macOS Mojave 10.14.1

Please follow these steps below in order to download macOS Mojave 10.14.1:
Check if the Mac system is compatible with macOS Mojave 10.14.1
Windows 8 apps free download for pc. The user should check if their Mac model is compatible with the macOS that they are about to download and install. These are the models that are supported:
- MacBook(2015 and onwards)
- MacPro(2013 and onwards)
- MacBook Pro(2012 and onwards)
- MacBook Air(2012 and onwards)
- iMac(2012 and onwards)
- Mac Mini(2012 and onwards)
Backup the system
The user should backup all of the important files that they may be afraid of losing. Time Machine is an excellent inbuilt feature that can help the users take care of this. The user should connect their system with an external storage device and then let Time Machine backup their files to this location. If the user’s original files are ever deleted or gone, they can use their backup files. DropBox, iCloud and OneDrive are also great alternatives to Time Machine.
Ensure that a strong Internet Connection is present
A lot of time is required to download and install macOS Mojave and the user should be thoroughly prepared for this. They should ensure that their Mac is connected to a strong Internet connection so that there are no interruptions in the download or installation of the macOS.
Download the macOS
The user can now download the macOS from their App Store.
Allow the Installation process to take place
The user will be prompted to begin the installation process once the download has been completed. The instructions displayed by the installer should be carefully followed and completed accordingly by the user. It is recommended that the user perform the installation in the evening so that the process can get completed overnight. They should ensure that their Mac’s lid is not closed and they should not enable the Sleep option either.
Enjoy the macOS for yourself
The user should patiently wait for the installation process to get completed( this process may take a very long time). Once it is done, the Mac will be restarted. After this, the user will be able to enjoy using and experiencing macOS Mojave 10.14.1 themselves.
If you have not tried out the macOS Mojave 10.14.1, we strongly suggest that you give it a try. Apple has done an incredible job of trying to improve upon the previous macOS High Sierra version. There has been a massive influx of new features in this version that we are sure you would love to try out.
Please peruse this article thoroughly before you update your Mac’s current OS to macOS Mojave 10.14 so that you will not miss out on any important information that you may require. We are so happy that we got the opportunity to be able to help you through this article. Please do reach out to us in the comments section below if you have any queries and we will help resolve them for you.
Mac OS Mojave 10.14.1 ISO & DMG Files Direct Download
Mac Os X 10.13 Download Apple
The macOS Mojave was the fifteenth release from Apple for their macOS family and was made available to the public on the 24th of September in 2018. The macOS Mojave 10.14.1 (the updated version) was released on the 30th of October of the same year with a couple of new updates.
Apple Mac Os 10.13 Downloadd Free
Price Currency: USD

Mac Os X 10.13 Download
Operating System: Mac OS Mojave 10.14
Application Category: OS
4.8

Sega Saturn Mpeg Rom File

Let’s face facts:Saturn titles are amongst the most expensive retro games around, and unless youwere keen to collect them when they were current or shortly thereafter, moneybecomes a huge factor. That said, thereare hundreds of worthwhile gameplay experiences just waiting to be played onSaturn, and there are several methods to play these games today outside oforiginal discs. Primarily this boils down to emulation + disc images, anoptical drive emulator (ODE) which replaces the Saturn’s physical CD drive witha small board that accepts SD cards instead of discs, or the most commonmethod: burning discs to play on real hardware. This article serves as acomprehensive guide on how to successfully verify, test, and burn Saturn DiscImages on Microsoft Windows Platforms.
Before we begin, weneed to acknowledge the obvious: burning discs is frowned upon by the industryas it almost always involves some level of piracy. Legally, one is onlypermitted to make copies of titles they already own. That said, with theSaturn, or any retro platform that is no longer officially supported there isno way to simply buy games in such a manner that the profits make their wayback to SEGA. Especially with the Saturn, there is no Virtual Console or PSNStore, etc. where one can buy digitally and download to play on their favoritemodern device. Gamers intent on having a more rounded Saturn experience mustresort to alternate methods of playing the games.
In this in-depthguide, we dive into the process of correctly burning reliable Saturn discs.
Sega Saturn Rom Collection By Ghostware Item Preview 1 Sega Saturn Magazine 01 (November 1995)(UK).cbr. 2 Sega Saturn Magazine 02 (December 1995)(UK).cbr. 3 Sega Saturn Magazine 03 (January 1996)(UK).cbr. 4 Sega Saturn Magazine 04 (February 1996)(UK).cbr. 5 Sega Saturn Magazine 05 (March 1996)(UK).cbr. Sega Saturn Rom Collection By Ghostware Item Preview 1 Sega Saturn Magazine 01 (November 1995)(UK).cbr. 2 Sega Saturn Magazine 02 (December 1995)(UK).cbr. Aug 28, 2020 The Sega Saturn was the second released Sega console which used CD-ROM to distribute its games, one of the benefits of the CD-ROM format is many times more space than a cartridge. One of the downsides compared to cartridges however was the slower loading times as reading from a CD is much slower than reading from a ROM chip. Welcome to cdromance BIOS section. Over here, we have a great selection of console BIOS files to use on emulators like the Dreamcast Bios for Reicast on Android devices and many more. These BIOS's can be used in any device, PC's, phones, tablets, RP (Raspberry Pi), so look no further you have found the best Continue reading 'BIOS Files'.
SOME THINGS YOU’LL NEED:
– A way to openarchive files. Common archive types include Zip,7Zip/7z, and Rar. On Windowssystems, we recommend 7z to handle allof these file types. It’s free and works great.
– A Hash file value checker. Any hash check program can be used; we primarily use CRC32 for our hash values.
– A Saturn Emulatorthat supports playing disc images. We normally use Yabause, but there are quite a few options available these days.
– A Burning Program.We strongly suggest CloneCD, and willeven provide Saturn disc read/burn profiles forCloneCD users. CloneCD is notfree, however it is highly reliable. We have yet to experience a bad burn usingCloneCD.
There are otheroptions of course, such as the much more popular (and free) ImgBurn. However, ImgBurn can’t burn RAW images, which means that an image has tobe altered to be burnt using ImgBurn ifthe image has Sub-Channel data in it. This limitation doesn’t really affectSaturn Games as the Saturn doesn’t specifically USE the Sub-Channel data in itsdiscs, but from a preservation standpoint, the data still exists and thereforean accurate image likely includes this data.
The originalPlayStation utilized this data often.
ImgBurn does have some limited CCD compatibility (it can handle a CCD image if there is no reference to the sub-channel data at all), but in our experience it is likely users will produce occasional bad burns, and that means your CD-R has become a handy coaster, or Frisbee. For this reason, we will keep recommending CloneCD.
– Optional: avirtual disc drive. Traditionally we use DaemonTools Lite as a virtual drive, however recent versions potentially tryto install spyware on your PC, so proceed with caution. You can also use Virtual Clone Drive from the makers of CloneCD. We use that as well, but not nearlyas often as Daemon Tools Lite as we haverun into compatibility issues with some 3rd party programs and Virtual Clone Drive.
Using a virtual discdrive allows you to “mount” a disc image as a drive which can then beused to test emulators like SSF that don’t permit the use of disc images.
– Optional, butHIGHLY recommended: CD Lens Cleaner.
Run a CD LensCleaner through your Saturn before attempting to play burns.
– Optional: Saturn Region Patcher 3.0 Gold
We do not recommend patching the region on game images. Instead, you should install a region switch or Universal BIOS, or just get an appropriate Region Lockout Bypass cart (Action Replay, Saturn Gamer’s Cart, Satellite, etc.). But, if you can’t/won’t get a cart, you can’t/won’t install a region lock bypass modification, and you INSIST on patching the region, you’ll need the tool linked below.
Please, do NOTspread region patched images around. The tool is very easy to use and regionpatched images have hashes that differ from the verified hashes at Redump, sothere’s not really any good way to ensure the patch was applied correctly or tocheck a patched image for data integrity.
– Optional: Pseudo Saturn Patcher and Compatibility List.
While Pseudo Saturn is FANTASTIC, it is not 100% compatible with every game out of the box. Some games, such as Batman Forever: The Arcade Game (US) requires a compatibility patch to allow it to work. Other games (Panzer Dragoon Zwei/Saga) *WILL* work unpatched on MOST Saturns, but you need to use the JHL loader (0.832) instead of the CWX loader (0.831). If it doesn’t work for you, use the Pseudo Saturn CDPatcher and try again. Both the patcher and the list can be found here:
THE GUIDE
We have tried towrite this guide in as much detail as possible, to ensure you have a goodexperience with burning Saturn discs.
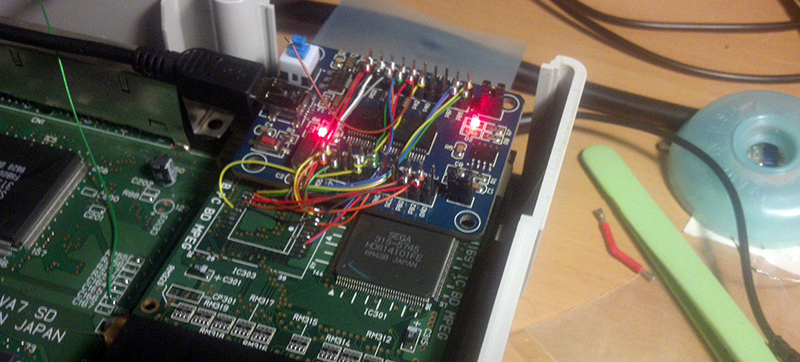
1. Always obtain your images from verified sources, or sources that have hashes for you to review.
This can mean you make your own images, obtain them from someone who knows how to properly and accurately make the images, or check images you get against a database hash like Redump. We cannot recommend you simply go to a random ROMs website like emuparadise. The goal for those sites has little to do with data preservation or accuracy. We have seen the same image with different titles, all broken.
2. Stick with one type of image (at least until you get consistently successful results)
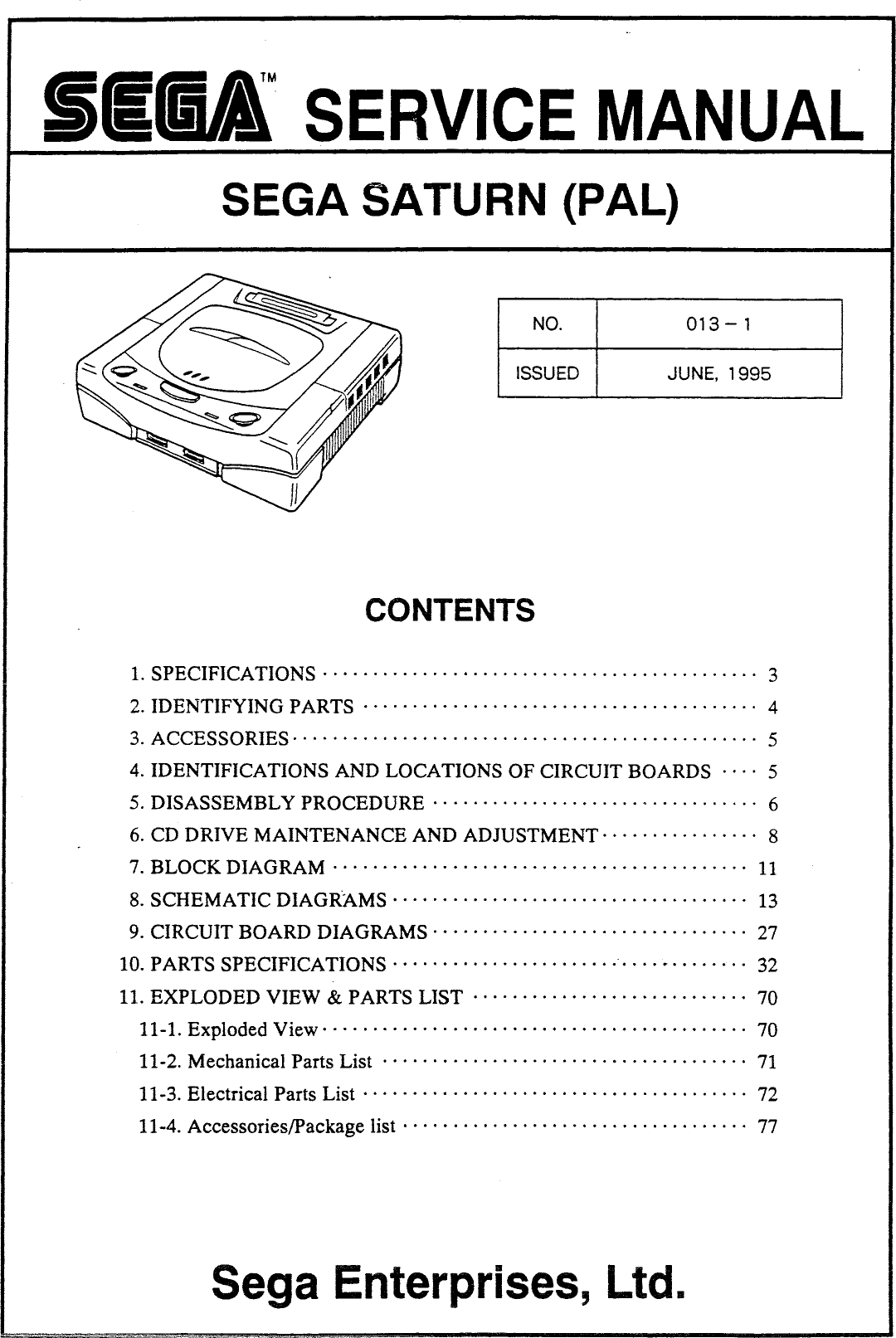
To start, we recommend CloneCD (CCD+IMG+SUB) to obtain burnt discs that are more closely accurate to the original disc and because it puts all tracks into a single file. Others use BIN+CUE. Redump’s images tend to be in BIN+CUE if they go public, but our goal is to maintain a CCD+IMG+SUB version of every file eventually.
Whatever you use,stick with it once you get it working. Variables and changes are a great way tointroduce uncertainty in your burns.
Once you’ve gotten afew burns done with this image type, you can consider setting up a differentburning program for different images, but follow the same steps!
3. DON’T USE “ISO+XXX” OR “YYY+MP3/WAV” IMAGES!!!
These images aresimply no good in the modern age and should be wiped from existence UNLESSthere is no other image for that game. The ISO format can’t even handle certainkinds of Saturn images at all, and MP3s are compressed so you lose dataregardless of how much it “sounds” like the original. Avoid like theplague!
4. Download and Extract Your image
Wherever you gotyour image from, it is probably in an archive format such as RAR, ZIP, or 7Z.You’ll need to use a program to extract the files from the archive. Make sureyou extract them all, and it is best to keep all the files from a singlearchive in a single folder, also separate from any other files.
Picture 3 shows aBIN+CUE image in 7z.
Picture 4 shows a CloneCD Image in 7z
Picture 5 shows the CloneCD image extracted to a folder in windows
If you get an errormessage from your Archive program, STOP and re-download the image, thisgenerally indicates a corrupted download. If it continually happens, contactthe group or person who provided the file and let them know what you’reexperiencing.
5. Open up the Redump listing for the game you’re working with
If you can’t find agame, it may not have been dumped yet, or you might be looking at the wrongregion or system option.
For this example, I (Ke) used Batsugun, found at http://redump.org/disc/5043/ (Picture 6)
6. Verify the hash of each appropriate file in the archive
If you have a BIN+CUE image, you’ll need to verify every single track against the appropriate hash value. This means putting each track into “Hash My Files” and comparing the appropriate hash value against the list of hash values in Redump for those tracks. (Picture 7)
If you have a CCD+IMG+SUB image, you only need to worry about the IMG file. You’ll compare the CRC-32 hash of this file against the value on Redump indicating TOTAL CRC-32 (Picture 8)
If all the hashesyou check match up, you can rest assured that your image has been verified asnot corrupted and unaltered, so you can eliminate the possibility of a“bad disc image” from potential problems.
If you run into anerror or mismatch for any hash check, re-extract the image and check again.
Note: Ke hasconverted the entire 2018 Redump Saturn archive into CCD+IMG+SUB from theBIN+CUE images, but has NOT as of yet verified all those image CRCs, so keepthis in mind if testing a CloneCD-based image Ke makes available. It takes aLONG time to properly verify 2050 disc images if it isn’t being done with somelevel of automation .
7. (Optional but VERY recommended) Test Image in an emulator
Most modern emulators will take an image file straight. Some (like SSF) will only work with a disc drive. Find what works for you. Emulator selection and setup is out of scope for this guide, but there are plenty of helpful Saturn community members.
Once you have yourchosen emulator functional, load up your disc image as appropriate and see ifthe game launches.
If the game launches, you can rest assured that the image as provided will work with Mod Boards/Mod Chips. If the image was in CloneCD format, you can also rest assured that the image will work with Rhea/Phoebe as is.
8. (Optional) Check Pseudo Saturn Compatibility Chart to see if the game you’re working with has any notes/Known issues
The Pseudo Saturn Compatibility Chart shows fairly good information about the compatibility of various games with Pseudo Saturn. Check here to see if the game you’re working with needs to be Pseudo Patched to be used on your Pseudo Saturn cart.
9.Panzer Dragoon Zwei/Saga Only
For ages, Panzer Dragoon Saga has been considered difficult to successfully play off a burnt disc without a mod board / modchip.
The game isn’t compatible with Pseudo Saturn 0.830 or 0.831, also known as the CWX Loading Methods.
One thing that is not very well documented is that Panzer Dragoon Saga (US) is completely compatible Pseudo Saturn 0.832 (implemented in Pseudo Saturn Kai as the JHL Loading Method) on MOST Saturn system revisions, even without patching. This was confirmed multiple times by Ke from the moment 0.832 hit the scene, and again when Shiro researched Panzer Dragoon Saga for Claire’s article last year.
However, we are not in possession of every single motherboard revision out there, and reports have come in that some motherboard revisions don’t allow you to play this game using Pseudo Saturn How to download mac apps. without applying the Pseudo Saturn Compatibility Patch first.
http://ppcenter.webou.net/pskai/#download(Pseudo Saturn CDPatch)
Note that despite Saga not ALWAYS requiring a patch to play on JHL/0.832, there is code within the game that detects what kind of cart you have in the system, and if the detection check “fails”, your cart is coming back as incompatible with the game. Generally speaking this check was to detect cheat devices and to detect carts that might allow for piracy. You can by pass this check using a game shark/action replay code, or you can attempt to patch it out using one of the Panzer Dragoon Saga Cheat Detection Bypass patches out there. Just be sure to only use that patcher if you absolutely have to, and only use such a patcher on a verified image.
10. Burning Your Game
General Tips:
– Use media with agood reputation. A brief Google search should help you determine whether yourchosen brand has a quality reputation.
– Until you’vegotten a few successful burns under your belt, shut down ALL other applicationsbefore you start your burns, initiate the burn as slowly as yoursystem/media/burner/burning program will allow, and don’t do anything until theburn completes. As you get more successful, you’ll find that modern computerscan handle multitasking quite well, but you don’t want to introduce variablesinto your process until you’ve knocked a few out.
– Until you’ve gotten a few successful burns under your belt, and again whenever you run into a problem, you should try to verify your burn. When the burn is done, if your burning program offers it, do a verification of the burnt data. CloneCD doesn’t allow for this but ImgBurn does, so keep that in mind. Since ImgBurn can’t deal with sub-channel data anyway, we will sometimes burn a Verified CCD+IMG+SUB image with CloneCD and then have ImgBurn do a verification check against a BIN+CUE image of the game that is a verified match.
– If you DON’T have a method to play imports on your system such as a Universal BIOS mod (aka “region free” bios, though that is not a correct term for what that mod does), Region Bypass Cart (Saturn Gamer’s Cart, Action Replay, Satellite, etc.), or a Region Switch, you’ll want to patch your image with SRP 3.0 Gold, listed in the opening section. Remember, please don’t share region patched images.
Pictures 9 through 16 show how to burn using CloneCD, including what settings you should use for Saturn games. Ke has created a profile that you can add to your CloneCD installation that will have these settings ready to go for you.
11. (Optional) Test the burnt game with an emulator
Test the burnt gameusing the emulator you might have used in step 7 above. If the emulator playsit, you have made a burn that should work with a real Saturn using a modboard/mod chip, provided you used good media and have a solid working laser inyour Saturn.
12. Test the burn with your system

If you have a modboard, all you need to do is pop the disc in the system, close the CD lid, andpower the system on. Most mod boards have a feature that disable the mod boardif the system is powered on with the disc lid open, so be sure to have that lidclosed before powering the system on.
If you are using Pseudo Saturn / Pseudo Saturn Kai, be sure to update to the latest version of the firmware, or the firmware compatible with the game you’re working with (see notes on Panzer Dragoon Saga and the Pseudo Saturn Compatibility List).
Unless you’re trying to use the Netlink modem cart with a burnt Netlink compatible game, I suggest Cafe-Alpha’s Pseudo Saturn Kai firmware as a firmware base as it is much more full featured than the original Pseudo Saturn releases. You’ll need the Kai Lite firmware for carts based on the Action Replay, but can use the Kai Full with the Saturn Gamer’s Cart.
If you are trying to use a Netlink modem cart and a burnt Netlink game on a retail Saturn, then I’d suggest the original Pseudo Saturn 0.831 firmware instead of any of the Kai variants. While the originals are lacking in features compared to the Kai versions, the originals have a very easy to identify stop point that allows you to “hot swap” the pseudo flashed cart for the Netlink Modem before loading the game. We DO NOT recommend this in general as hot swapping can damage your Saturn, but we have performed this ourselves repeatedly with retail systems and have never noticed issues or detrimental effects. On debug/dev kit systems, there is additional power going to the cart slot which will cause the system to reboot if the cart is swapped, so avoid that.
Sega Saturn Mpeg Rom File Converter
Ideally, if you’re planning to use the Netlink, we recommend you invest in a mod board instead of Pseudo Saturn.
13. Troubleshooting Questions
1. Have yousuccessfully burnt discs, and then played those same burnt discs with yourcurrent setup (computer, operating system, burner, media, burning program,burning speed, Saturn, backup boot method) in the past?
2. Did you get yourimage from a known reliable and/or verifiable source?
3. Did you verifyyour image’s hash value(s) before attempting any patching?
4. Did the unpatchedimage work in an emulator?
5. Is the game knownto be compatible with your method of playing backups? If yes, is a patchrequired for that game to use that backup playing method?
Realmac software rapidweaver web design software for mac pc. 6. If you applied apatch to the image, did the patched image work in an emulator?
7. Did you use mediawith a good reputation for quality?
8. Did you quit outof all other programs before burning?
9. Did you burn asslowly as possible for your media/system/burner?
10. Did you makesure to not do anything else while burning (troubleshooting only. If you aren’ttroubleshooting, you don’t need to be in this section anyway, right?)?
11. Did you allow the burning program to verify the data burnt to the disc (CloneCD can’t on its own, but there are other tools out there)?
12. Does the burntdisc work in an emulator?
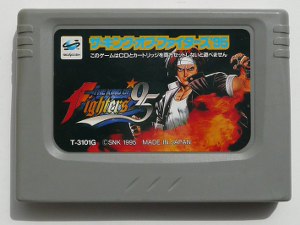
13. Has your Saturnbackup solution ever worked properly? If yes, can you boot other backups still?
14. Did you recentlyclean your Saturn laser pickup lens?
15, Have you triedthe same disc in a Different Saturn using the same backup solution?
16. Have you triedthe same disc in a Different Saturn with a DIFFERENT backup solution?
14. Addendum:
“Why should iuse CloneCD/CCD+IMG+SUB instead of ImgBurn/BIN+CUE?”
For Saturn, many inthe preservation community argue that the Saturn does absolutely nothing withSub-Channel data, so whatever data is in the Sub-Channel is essentiallyuseless.
Register now to download the latest demo of Redshift. Your coffee breaks are about to get much shorter. Redshift 2.6.23 crack mac. Prior to purchasing Redshift, we encourage all interested customers to try the Redshift demo version to ensure system compatibility and experience Redshift's amazing performance.
As apreservationist, Ke believes that the closer we come to an accurate backup ofan original disc, the better off everyone is in the end, regardless of if theoriginal system reads that data or not. Accuracy is important.
Ultimately, we donot dislike BIN+CUE so much as we dislike having multiple disc image formatsfor the same disc.
Deunan (creator of the GEDMU and Rhea/Phoebe Optical Drive Emulators) and Ke had a conversation about trying to find a disc image option that was universal across all platforms (Mac, *NIX, and Windows), and why Rhea/Phoebe didn’t support certain image types. He came away from that conversation with a LOT more understanding, and dropped the hope of a universal format. He was already a CloneCD fan/user, but now He appreciates it so much more.
Also, whileCCD+IMG+SUB isn’t compatible with Mac or *NIX machines, those systems can oftenrun Windows in a virtual environment or via dual booting, which solves thecompatibility problem.
Here are some snippets from that conversation; all Deunan’s words but lightly edited for spelling or context, as well an an excerpt from his blog post in 2014 about supported formats for Rhea:
In short:
– CUE is a brokenformat, too simplistic for anything but the most common audio CDs
– it’s broken evenfor CDs with seamless audio tracks, it gets worse with games
– recentnon-standard extensions tried to correct that by adding missing TOC data
– but many programsdo it their own way, or not at all, plus you still can’t have subcode (EDIT:aka sub-channel) data attached to the image/tracks anyway
– older dumps don’thave that extended data and will break anyway, I’ve already had “bugreports” for games converted from CUE to other formats
– and there are CUEswith compressed audio tracks…
Sega Saturn Mpeg Rom File System
Let me just say thatALL current ripping methods are equally flawed, it’s just some programs atleast try to get it as good as possible given the limitations of typical PChardware.
In reality there isno such thing as rip accuracy, but if you use the same software, hardware andmethod you should end up with a bit-exact result. So while you can verify therip, it does not really mean this one particular variant is the “correct”one and all others are not. In general though, most discs mastered mid-90’s orlater should be well enough structured to produce identical results even withdifferent Hardware and tools.
For personal usagejust stick to general ripping guidelines like correct settings, non-brokenformats, and that’s it. All those efforts to re-rip all games for a givenplatform every few years are just wasted time mostly, unless the storage formatis really superior. This would be the case when replacing CUEs with CCD or CDIfor example, but CUE to CUE is at best fixing the really broken dumps, but notreally improving the quality of the rest.
CloneCD is pretty good when it comes to CDs. I’m also a fan of open formats but unfortunately all of them are rather lacking. Well it’s not really a surprise the best solution is the one you have to pay for 🙂
So, stick to CCD Iguess. I’m probably going to as well as CDI is discontinued and will eventuallyfade into obscurity as it stops working with more modern OSes and HW.
– Deunan
Sega Saturn Sonic R Download Rom
– Source: Privateemail conversation
After some initial issues Rhea has been upgraded to V1.5 and works pretty stable now. As in boots everything I throw at it. Which brings me to subject at hand: Many of the existing images of Saturn CD-ROMs are pretty poor.
I’m going to have toput a line somewhere because I don’t want users to complain about the devicenot working properly when the problem is with the dump itself. Only certainformats will be supported, and as always I urge you to make your own dumps.It’s not that complicated, Saturn discs can be read on a normal PC drive – nospecial hardware needed.
Supported formats:
CDI – Preferred.Needs to be either RAW or (optional) RAW+SUB format. CDIs include pregaps(index 0 parts of the tracks) by default which is good.
MDS/MDF – I’ve justadded preliminary support for this format. The only few images I have are inRAW+SUB format, but just RAW should be fine as well.
BIN – Only forsingle track images, needs RAW sectors. Since I haven’t seen a single trackgame yet I don’t think this one will be of much use – perhaps for homebrew.
I’m looking atCCD/IMG format now, I might add some limited support for that as well sinceit’s popular. Please note that having the dump in supported format doesn’t yetmean it will definitely work – the file format is one thing, and the quality ofwhat’s actually inside is another.
Not supported:
CUE – Anything thatdepends on CUE will not work. I’m not even talking about ISO+MP3 tracks, whichare the worst, but the whole format in general. There are many ad-hocextensions to the so-called standard CUE, and the tracks can be multiple files,single file, RAW or MODE1, even compressed via MP3 or FLAC, or converted toWAVE instead of raw audio data. Single file “pregaps” are just zero-filledspace even for data tracks. And to add insult to injury the MSF address of eachtrack is always +2 seconds, pregap or not, because it was more funny this way.
Some CUE based dumpscan be converted to a more sane format and will work. I’ve tested it bymounting the image in Daemon Tools Lite and re-ripping it with DiscJuggler toCDI. For some reason DJ doesn’t see WinCDEmu virtual drives, so that’s why DT.That’s just one possible option, and obviously if the dump was bad to beginwith this will not produce a working image. But might be worth a try if yourdisc is damaged and unreadable and CUE is all you’ve got.
It’s always best tostick to properly made dumps, even if these need to be converted you know thedata is good. I can’t guarantee correct operation otherwise.
– Source: Deunan’sGDEMU Blog,
– https://gdemu.wordpress.com/2014/10/08/saturn-rings/
You’ll note that myemail conversation with him happened about a year after his blog post, as hispreference for CDI had shifted to a preference for CCD, which was FANTASTICsince i haven’t had a working DiscJuggler setup in years.
15. Addendum 2: But I use Mac/*NIX and can’t burn CCD+IMG+SUB files!!!
Dual Boot or run aVirtual Machine that allows access to your disc burner.
16. Conclusion
Burning Saturn gamesdoesn’t have to be difficult, but one of the most famous lines in datamanagement has always been “Garbage In, Garbage Out”. This stressesthe fact that computers can only do so much with the data provided to them, andonly with the tools available to it. If you provide an unverified disc image toa burning program, you introduce the possibility of problems with the imageitself, on top of any potential issues with your computer, your burningsoftware, your burner, your backup playing method, or your Saturn laser itself.
Systematicallyeliminating the possibility of a point of failure makes it that much easier totroubleshoot when something goes wrong.
This is a LONG andTEDIOUS process, designed to root out problems you might have with a burnt discbefore it gets to the burning stage. Once you’ve done this process 4-5 timessuccessfully, the amount of steps can be reduced, but the moment you run into asnag, zero back to this guide and follow it step by step to see where yourpoint of failure actually is.
Above all else,remember… You MUST play SEGA Saturn!

Bangla Hsc Math Books

Bangla Pdf Book download, SSC-HSC Shortcut Note, Hons, Circular, Results, bangla book pdf. HSC Math 1st, 2nd paper all book and notes Pdf Download Tags. Academic Book. উচ্চ মাধ্যমিক এর গণিতের সকল বইয়ের পিডিএফ লিংক নিচে দেওয়া হল. HSC Mathematics First Paper & 2nd Paper বই দরকার।।অনুগ্রহ পূর্বক আপলোড করবেন।। ১৮ নভেম্বর, ২০১৪ ১১:৪৯ AM.
If you are searching for the HSC books, I can assure that you will get the PDF copies of class 11 books here. Bangladesh Education Board is also started sharing PDF books like General Board. Collect all the PDF books of HSC class 11 books from here. I hope these PDFs will help you with your studies.
Book Details:
Adobe premiere pro cc for mac os x 10.7.5. Adobe Premierre Pro CC is an industry-leading video editing software, you can edit virtually any type of media in its native format and create professional productions with brilliant color for film, TV, and web.Easier collaboration with Team ProjectsCollaborate and share sequences and compositions in real time with Adobe Team Projects (Beta).
Windows 8 apps free download. Use the media creation tool (aprx. 1.41MB) to download Windows. This tool provides the best download experience for customers running Windows 7, 8.1 and 10. Tool includes: File formats optimized for download speed. Built in media creation options for USBs and DVDs. Optional conversion to. Free APK APPS Download For PC Windows.Free and safe download.Download the latest version apk free download For PC,apps for pc and full version apk for Android. Download Windows apps for your Windows tablet or computer. Browse thousands of free and paid apps by category, read user reviews, and compare ratings. Free Apps For PC Windows 7,8,10,Xp Free Download. All Free PC Apps and PC Games are downloadable for Windows 7, Windows 8, Windows 10 and Windows xp.Pcappswindows.com is one of the best places on the Web to play new PC,Laptop games or apps for free in 2019!Download free apps online.Apps for PC are free and safe download.Download the latest version apps apk games for PC.Download APK/APPS.
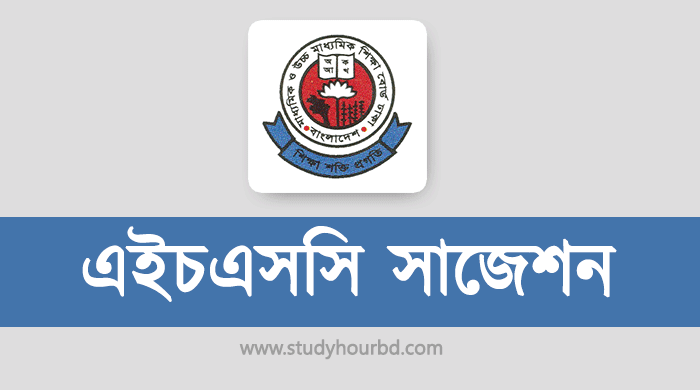
- Download your necessary books.The motto of Bangla Books PDF. For keeping that commitment, Bangla Books PDF is going to add all NCTB books to its books store for download. In this post, you will get access to download all books of class 1-10 provided by NCTB.
- এইচএসসির পিডিএফ বই দেখব যেগুলো কিনা ইন্টারনেটে সহজে খুজে পাওয়া যায়না ।- HSC book download pdf ডাউনলোড করুন ।একাদশ-দ্বাদশ শ্রেণীর সকল বই ডাউনলোড করে নিন ।.
- Nctb books of class 9-10 bangla version 2018, nctb books of class 9-10 bangla version, class 9 physics book pdf bangla version, nctb books of class 9-10.
- Book Name: HSC Books | Class 11 Books
- Genre: HSC Books
- Category: TextBook
- Published: 2020
Class 11 Bangla Book
- Size: 17 Mb
Class 11 Bangla Sohopath Book
- Size: 32 Mb
Class 11 English Book
- Size: 10 Mb
Class 11 Higher Math Book
- Size: 12 Mb

Class 11 Higher Math Solution Book
- Size: 26 Mb
Class 11 Physics Book
- Size: 06 Mb
Class 11 Chemistry Book
- Size: 25 Mb
Class 11 Biology Book
- Size: 04 Mb
Class 11 HSC Statistics Book
- Size: 07 Mb
Class 11 Management Book
- Size: 10 Mb
Class 11 ICT Book
- Size: 07 Mb
Class 11 Accounting Book
- Size: 13 Mb
Similar titles
Collect HSC books from Checking for pending restart. here. You will get the PDF copies of class 12 books here. This article is about the Alim PDF books download. Ebooks or PDF books are really helpful to students. Because most of the students have a mobile phone nowadays. Students can download the PDF books and keep the books in their phones and could read anytime anyplace.
Book Details:
- Book Name: HSC Books | Class 12 Books
- Genre: HSC Books
- Category: TextBook
- Published: 2020
Class 12 Bangla Book
- Size: 17 Mb
Class 12 Bangla Sohopath Book
- Size: 32 Mb
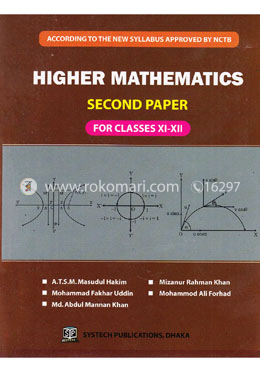
Class 12 English Book
- Size: 10 Mb
Class 12 Higher Math Book
- Size: 12 Mb
Class 12 Higher Math Solution Book
- Size: 26 Mb
Class 12 Physics Book
- Size: 22 Mb
Class 12 Chemistry Book
- Size: 28 Mb
Class 12 Biology Book
- Size: 04 Mb
Class 12 Business Policy Book
- Size: 08 Mb
Class 12 Management Book
- Size: 10 Mb
Hsc Bangla 1st Paper
Class 12 ICT Book
Math Books For 4th Graders
- Size: 07 Mb
Class 12 Accounting Book
Hsc Bangla 1st Paper Class
- Size: 06 Mb
Bangla Hsc Math Books 2017
Similar titles
